Inheritance for Common Fields and Spring Audit
Nov. 03, 2022 · 5 min read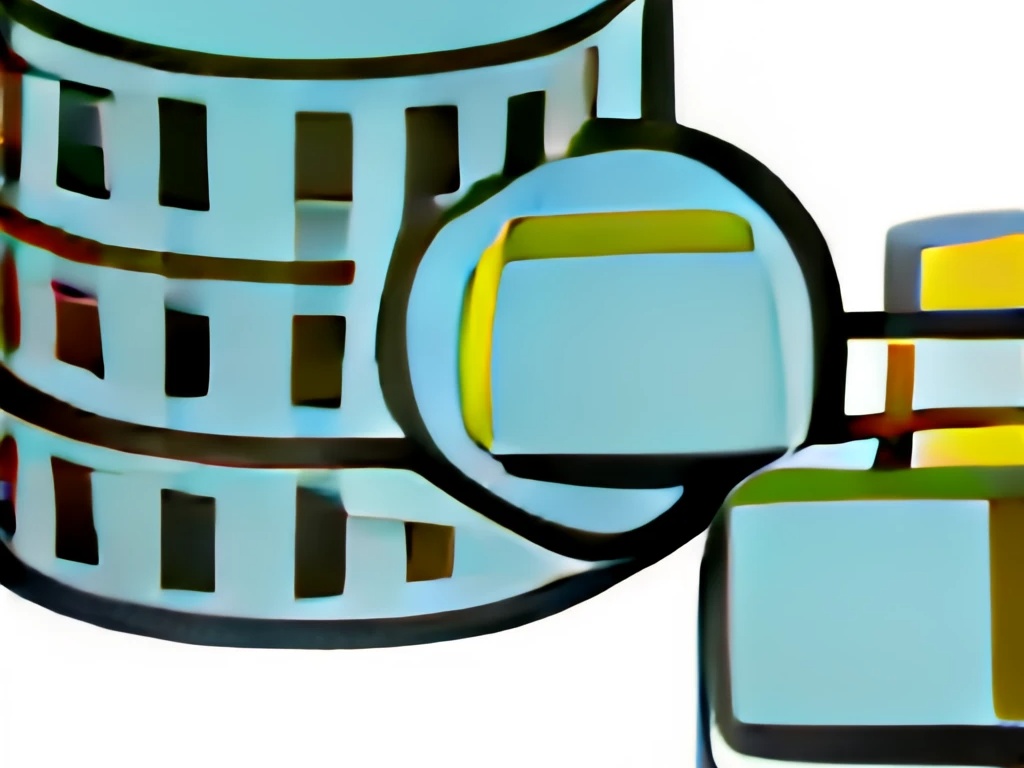
In this article, we are going to focus on common fields and how it can affect our application if we do not plan ahead of time.
Introduction
The Common fields are fields that are similar and present in two or more classes in Java.
Declaring common fields for each class in a scalable project could be cumbersome as it makes you repeat yourself and could make lengthy codes.
But how about dealing with timestamp fields? Is there another way we could implement it seamlessly? Let’s find out.
DRY Principle and Inheritance
The DRY principle is a technique that most developers employ, particularly in Object Oriented Programming. DRY is an acronym that stands for Do Not Repeat Yourself. It simply means you should not write the same code over and over again.
You are doing the same thing twice if your application has similar fields or codes spread across numerous classes. As a result, you are not following the principle. It will be challenging to manage and maintain, and if there are any changes, you will need to update it everywhere you write it, taking time away from everyone.
Breaking down your code and logic into smaller yet reusable components can help to prevent this worst-case situation. It is better to have less and simpler code because it will take less time and effort to maintain, will be easier to update, and will be less likely to include bugs.
A way of applying the DRY principle is to make use of inheritance. Inheritance means that a class can inherit all the properties from another class. With that being said, we remove code duplication and we are not repeating ourselves, thus it allows us to reuse the code.
Spring Audit
Now that we are aware of how to reuse common fields rather than rewriting them for every class. With regards to timestamp fields, we are moving forward together with Spring. Introducing Spring Audit, every change we make to our persistent data is tracked and logged by this feature, which also tracks and logs all insert, update, and delete activities. Additionally, it is necessary for tracking user activities.
The first two examples use Spring Audit but have the same timestamp fields which cause lengthy code and not applying reusability.
According to the spring documentation, the AuditingEntityListener is a JPA entity listener for auditing information on persisting and modifying entities.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
@Entity
@EntityListeners(AuditingEntityListener.class)
public class Employees{
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int id;
private String lastName;
private String firstName;
private String email;
private String password;
@LastModifiedBy
@Column(columnDefinition = "bigint default 1")
private Long updatedBy;
@LastModifiedDate
private LocalDateTime updatedAt;
@CreatedDate
private LocalDateTime createdAt;
private LocalDateTime deletedAt;
// getters and setters
}
@Entity
@EntityListeners(AuditingEntityListener.class)
public class Holidays {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int id;
private String holidayName;
private LocalDate holidayDate;
@LastModifiedBy
@Column(columnDefinition = "bigint default 1")
private Long updatedBy;
@LastModifiedDate
private LocalDateTime updatedAt;
@CreatedDate
private LocalDateTime createdAt;
private LocalDateTime deletedAt;
// getters and setters
}
The following examples use inheritance. Notice that there is reusability of code and it is smaller and cleaner code to look at.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
@MappedSuperclass
@EntityListeners(AuditingEntityListener.class)
public abstract class Auditable {
@LastModifiedBy
@Column(columnDefinition = "bigint default 1")
private Long updatedBy;
@LastModifiedDate
private LocalDateTime updatedAt;
@CreatedDate
private LocalDateTime createdAt;
private LocalDateTime deletedAt;
// getters and setters
}
@Entity
public class Employees extends Auditable{
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int id;
private String lastName;
private String firstName;
private String email;
private String password;
// getters and setters
}
@Entity
public class Holidays {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int id;
private String holidayName;
private LocalDate holidayDate;
// getters and setters
}
Summary
This article focused on what are common fields and how to reuse them in order to apply DRY principle and also knowing Spring Audit which can give a big help for tracking and logging user activities in the database. In the example given, we had noticed the lengthy code and repetitive audit fields in the Employees and Holidays class respectively. If there is a change in the database implementation, suppose we are removing some audit field for all classes, which means we will waste a lot of time rewriting again for each class. So for that not to happen we then try to remove the repetition and encourage reusability by applying the DRY principle and inheritance.